What is MVC and Why Should I Use It?
The Model-View-Controller or MVC is a software design pattern that helps drive the design of architecture in an application.
It's a way of designing the structure of a system that separates application and business logic from the user interface.
The idea is you can separate your application into three major components. You have models, views, and controllers.
Models handle the data structures, logic, and rules in your application.
Views are responsible for presenting models and other requested data.
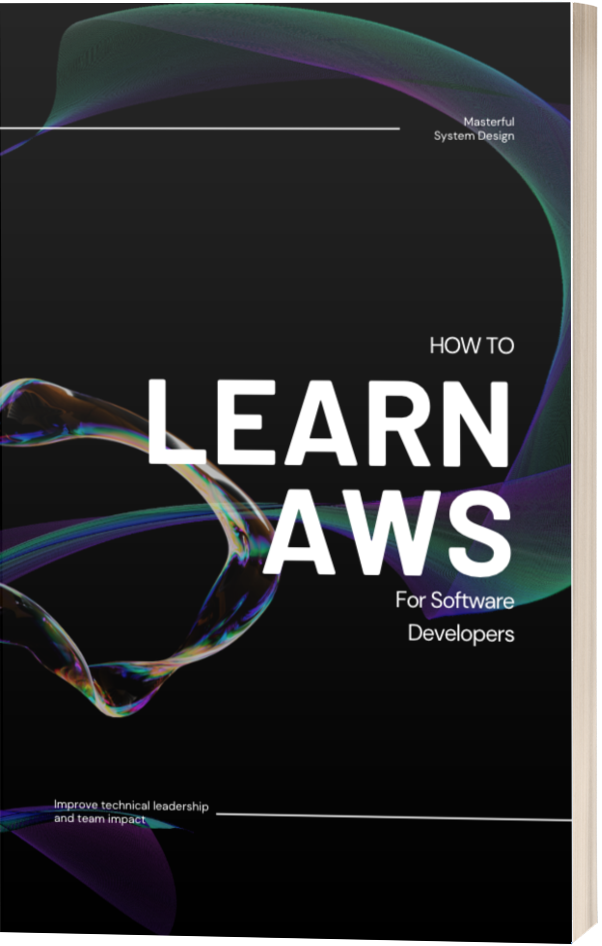
Controllers respond to input or requests and perform actions on your models.
Ruby on Rails, Laravel, and many other widely used web development frameworks have adopted this pattern.
How the MVC Pattern Works
I'm a huge advocate of Ruby on Rails and I think it demonstrates this pattern really well.
Within a Rails app, you will find 3 directories located at app/models
, app/views
, and app/controllers
.
When a person visits your website, their browser sends a request to your Rails app. The routes.rb
file will interpret the specific endpoint the visitor is requesting and point to a specific controller to handle the request.
The controller then performs its responsibility of handling this user input and pulls in the necessary models and business logic to satisfy the request from the visitor.
For example if a visitor is reading your blog, the controller will likely fetch a collection of blog posts. These blog posts would be defined as a model and the logic specific to finding the correct posts and pulling data from the database falls on the shoulders of the model.
Lastly, the view comes into play. The controller has now interpreted the request and fetched the models needed based on what the visitor is asking for. Now it's time to serve that content to user. The view will take in model data and render the actual HTML of our website.
The browser finally receives a response back including this HTML and renders it for the visitor to see.
And we're done. All of that happens in less than a second.
Benefits of Using an MVC Framework
When you use the MVC pattern you get to benefit from a set of conventions that make a lot of decisions easy.
Instead of asking yourself where a certain piece of code belongs, you just have to follow the predefined structure of MVC.
Let's jump into a few examples that show when you would want to modify the models, versus the views, and lastly the controllers.
When Do You Modify Views?
So, views are responsible for presenting information to the user.
Let's say we wanted to add a new widget to our website. It's purely cosmetic. Because of this I know we won't need to fetch any data from the database, so I don't need to think about my models.
Also, since we aren't fetching any data I know I don't need to make any requests so I can ignore my controllers.
All that's left are my views and I know views are responsible for handling the display of content. I can add my widget to the correct view and move on to the next feature.
Without following a pattern such as MVC, you might not be able to organize your application in such a structured way. As a result, it could take longer to think about and make organizational decisions and I would bet your application would get messy pretty quickly.
When Do You Modify Models?
We know models are responsible for handling data structures and logic in our application.
Let's build on our previous example and add some data to the widget we added to our view.
For this example, we can say our widget needs to render the current user's name and email.
We can assume this data is stored in the database.
In order to access it, we have to modify our models to read from the database and pull the user.
When Do You Modify Controllers?
Lastly, we know that our controllers are responsible for taking in requests from users and calling the right models to perform our business logic.
Our previous examples worked because we assumed there was already a controller and we added the widget to an existing view. If we needed to pass additional data our controller would be responsible for calling the models that contain that data and passing them to the view.
It's almost always the case that when you are creating a brand new page on your website, you will need to create a new route that in turn points to a new method in your controller.
When we follow the MVC pattern we can separate different areas of our codebase based on the feature we are building.
Since controllers take in requests from users, anything that deals with new requests or modifying existing requests will typically result in a code change in a controller.
Some Final Tips Before You Go
Even though Rails is an MVC framework, you can still technically write whatever code you want.
If you don't like models you could technically just keep all of your logic in your controllers.
And for views, you could also skip them altogether and just render raw HTML from your controllers. (I highly, highly advise you not to do this though!)
Ultimately, if you don't like this pattern, you don't have to follow it. That's the great thing about software development.
Frameworks and patterns are simply tools to help you and make things easier, but they're flexible and optional.
BUT if you do follow the MVC pattern, all of your organization and structural decision making will be easy. You don't have to think about it because there are predefined conventions you can follow and there's a correct answer for where to put something.
For example, the Rails community has a saying: "Skinny controllers, fat models".
Namely, keep your controllers small and light. They're only responsible for taking in requests and inputs. Don't put lots of if statements and logic in them.
Instead, add your application logic to your models where this stuff belongs.
Conventions like this can help drive more efficient development and make it easier for you and your team to grow and scale your codebase as your application grows.
You'll end up with much cleaner code.
Also, as new developers join your team they can find whatever code they need to because it's organized in a way that everyone agrees on and can understand.
Want to learn more?
Get on my email list and I'll let you know about new stuff I discover and write about. There should be a form at the bottom of the page.
I'm also active on Twitter where I talk about some other cool things.