A Quick Introduction to TypeScript
TypeScript is a programming language that adds a type system to JavaScript.
Traditionally, JavaScript is a dynamically-typed language. It uses primitive types like strings, numbers, and objects but it doesn't require you to declare these or use them consistently.
This means you can create variables and objects and their type can be inferred or changed later. On one hand, this is nice not having to declare the type of object or variable you are creating, but it can also hurt you.
Why Types are Awesome
When you use a type-system you get a few key advantages.
First, the compiler will be able to tell if you change the type of a variable or call a function on a variable in an impossible way.
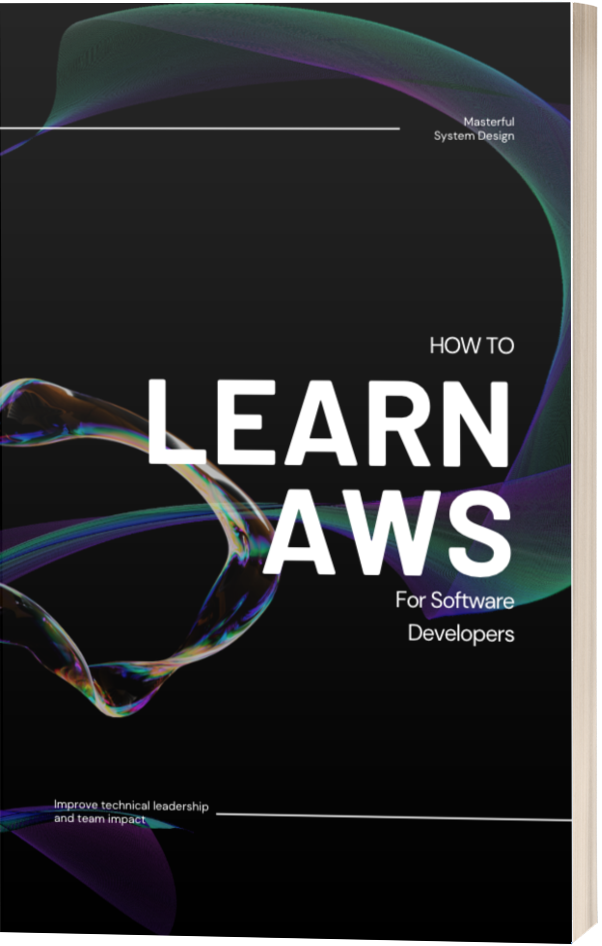
Let's look at an example of what can happen without a type-system:
a = true
b = 'hello'
c = b / a
It's impossible to calculate c
here, we can't divide a string by a boolean.
The problem is, we don't declare any types so we don't know what a
and b
are it's too late. We won't catch this error until we run our code and see it crash.
With TypeScript, things look a little different:
const a: boolean = true;
const b: string = 'hello';
const c: number = b / a;
We can tell the compiler what type each variable is which allows us to catch that we are trying to perform an illegal operation.
The first example doesn't use any type-checking and could be unintentionally released more easily.
Our TypeScript variant would fail and throw an error during compilation. So we would know our code is bad and fix it before it goes to production.
The main benefit of TypeScript is that it can highlight unexpected behavior in your code, lowering the chance of bugs.