Introduction to JavaScript Testing with Jest
Table of Contents
What is Jest
Jest is a super fast and easy to use test runner for JavaScript. It's also open-sourced and maintained by Facebook.
It's great for testing React code but really it can test anything written in JavaScript.
You write tests and Jest runs them for you. Jest also gives us other helpful tools like mocks and snapshots which help us write better tests too.
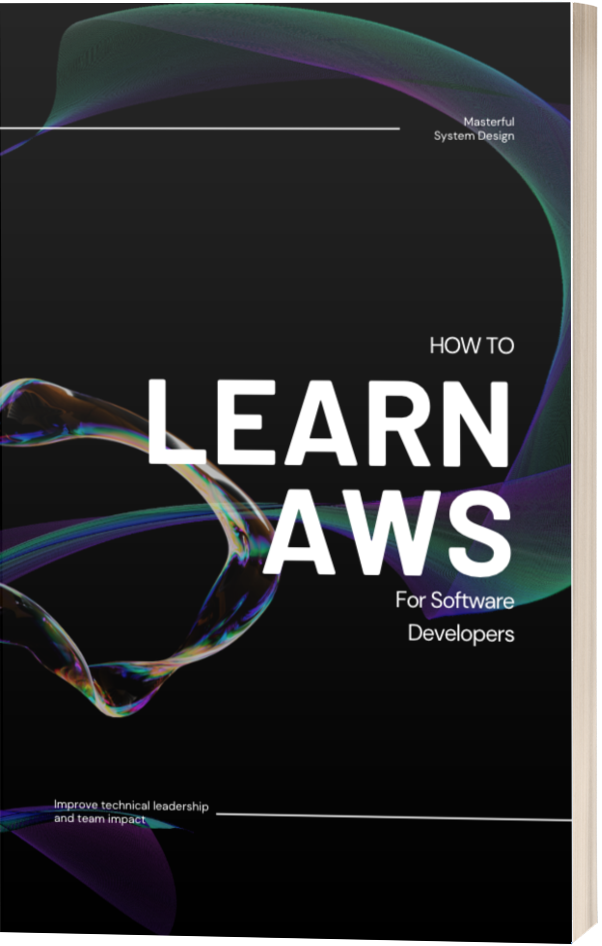
Installing Jest
You can install Jest using npm:
npm install --save-dev jest
or yarn:
yarn add --dev jest
And note the --save-dev
flag we use. We want to install Jest as one of our devDependencies in the package.json file. This is because we don't run tests after code is already in production, we do it before in our development environment.
Next, we need to add this line to our scripts in the package.json file:
{
"scripts": {
"test": "jest"
}
}
This lets us run npm test
and yarn test
.
Getting Started with Jest
The easiest test we can start with is a snapshot test.
These save the output of a particular component and check to see if that component has changed since the last snapshot.
This helps prevent unintentional changes and side effects when modifying code or components that are shared across components.
We can write our snapshot tests like this:
it('renders correctly', () => {
const tree = renderer.create(
<Component onPress={jest.fn()}>Do it</Component>
).toJSON();
expect(tree).toMatchSnapshot();
});
The it
function is an alias of test
and either can be used to create a test.
Our test runs this function that creates our component and saves it to a variable called tree.
We then assert that the component looks exactly like the last snapshot we took of it.
If it does then we pass! If not, we know that something unexpected changed our component. We'll know we need to fix this component before we release.
How to run our tests?
Now that we have some basic tests, we can run them with Jest.
The command to start your tests will depend on your package manager.
If you added Jest to the 'scripts' portion of your package.json then you can run your tests with npm test
or yarn test
.
Final Words
This guide should introduce you to Jest and help you learn the basics to write your first test.
Now you'll want to beef up your test coverage and write tests for your code.
We have a helpful guide on testing specifically in React and React Native here, coming soon. The same principles can be applied to other frameworks too.
You can reach me on Twitter if you have any questions or want to connect!