Naming Conventions for Concerns in Ruby on Rails
Naming things is hard.
It's the most popular joke in software engineering for a reason.
The two most difficult aspects of software development are caching, naming, and off-by-one errors.
As with most of the Ruby on Rails framework, you get the configuration of Rails with the flexibility of Ruby. So you can really name concerns however you want.
Let's talk about how to name your Rails concerns better.
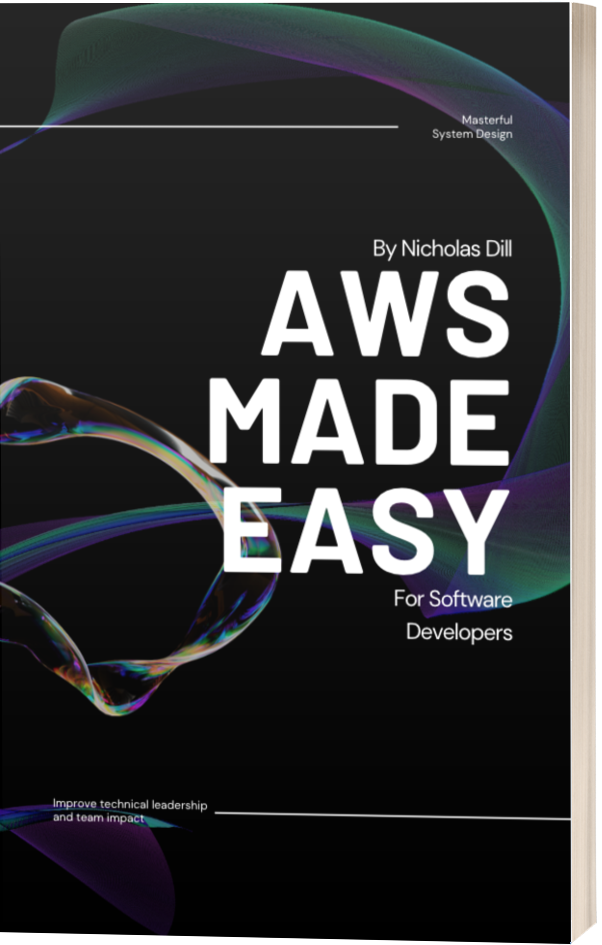
How to Name Concerns
There are a few common patterns you will find across most Ruby codebases, but one stands out as the clear majority favorite.
THE -ABLE NAMING CONVENTION
In short, describe the functionality a concern adds to other classes and append it with "-able".
If you have a concern that gives other classes access to avatars or profile pictures, you might name the concern Avatarable
.
It's best to name concerns based on the functionality they add to a class because this provides more context and makes the class overall easier to understand.
And if you're still not convinced, Rails source code even follows this pattern. It has a concern named Configurable that gives a given class some configuration logic.
DRAWBACKS WITH CONCERNS
One of the main drawbacks when it comes to using concerns is the fact that they provide access to methods and associations that aren't defined in the original models.
This can often be confusing or even frustrating.
Having clearly named concerns can help alleviate these drawbacks and give other developers a better idea of where these surprise methods are coming from.
Anyway, here are some more examples.
EXAMPLES OF -ABLE CONCERNS
You might have a few different models in your software that are each written by authors and as a result, need a lot of shared methods to manage authors.
Your blog posts have authors, your comments have authors, and even your testimonials have authors.
Instead of copy/pasting the belongs_to :author
association and any methods that are needed in all of these classes, you can extend a concern that makes that class "authorable".
And so you would create an Authorable
concern that could look something like this:
module Authorable
extend ActiveSupport::Concern
belongs_to :author
def author_name
author.full_name
end
end
Now regardless of if you are dealing with a BlogPost, Comment, or Testimonial model (assuming these are models that would use this concern) you can access any of the code within your concern.
Here's what that might look like:
post = BlogPost.find(42)
post.author_name
=> "Best Author"
comment = Comment.find(21)
comment.author_name
=> "Best Visitor"
testimonial = Testimonial.find(42)
testimonial.author_name
=> "Best Customer"
How to Use a Concern
Concerns are a type of "mixin" and are included in a model by calling include
, such as include Authorable
.
In the previous example models we used, it could resemble this:
class BlogPost < ApplicationRecord
include Authorable
end
class Comment < ApplicationRecord
include Authorable
end
class Testimonial < ApplicationRecord
include Authorable
end
And now any of these classes would be able to use the shared logic present in the concern.
Granted, just because each of these models is now "authorable", they would still need database migrations such that they could store the author id they are associated to. Don't forget that important step!
But otherwise, as you can see there's no need to be concerned - concerns are pretty straightforward!