Importing and Using Fonts With JavaScript
I ran into an issue where I was adding elements to the DOM and styling them purely with JavaScript, but I needed to change the font style.
The fonts I wanted to use needed a few extra steps in order to work since they needed to be imported from somewhere.
Here's how I was able to make it work.
Adjust a font style with JavaScript
First, we can add the style property to an element with JavaScript like this:
element.style.fontFamily = "Lato";
However, if we don't import the font this isn't going to do anything!
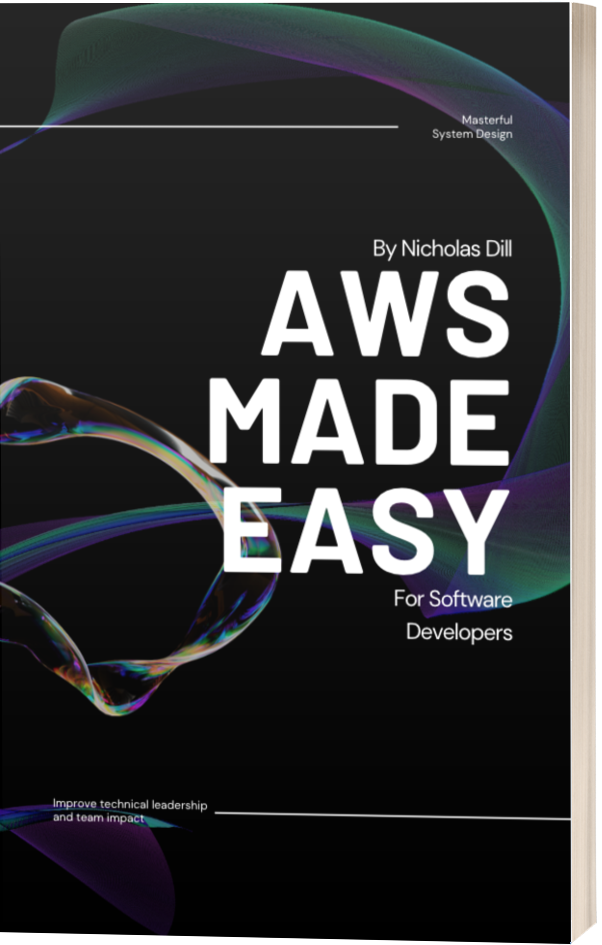
Import a font family with JavaScript
We can create and add DOM elements like divs and spans, but we aren't limited to just structural elements.
We can add <link>
and <style>
elements too!
Here's what it could look like:
var link = document.createElement('link');
link.setAttribute('rel', 'stylesheet');
link.setAttribute('type', 'text/css');
link.setAttribute('href', 'https://fonts.googleapis.com/css?family=Lato:300,400,700');
document.head.appendChild(link);
How does this work?
First, we create the element. We can use document.createElement('link')
to create the <link>
element we talked about earlier.
Next we need to add a few attributes to specify the attributes and where to import the font from.
Let's declare this as a stylesheet by setting the rel attribute to stylesheet.
link.setAttribute('rel', 'stylesheet');
Then we specify the type as CSS.
link.setAttribute('type', 'text/css');
This will let us link to the font family that we want to import for our text.
link.setAttribute('href', 'https://fonts.googleapis.com/css?family=Lato:300,400,700');
Last, we need to add this entire element to the DOM.
Typically, we import fonts and stylesheets in the head section of our page, so I'll append this new element to the document.head
.
document.head.appendChild(link);
All of this JavaScript will result in HTML that looks something like this:
<link rel="stylesheet" type="text/css" href="https://fonts.googleapis.com/css?family=Lato:300,400,700" />
And then any element that needs this font will be able to use it!
Again, since I needed to dynamically and programmatically change font styles, I added this font with some more JavaScript.
It looks like this:
var element = document.getElementById('banner-title')
element.style.fontFamily = "Lato";
Wrapping Up
This guide was inspired by a set of features I'm building for UserSurge.
It's one of a few projects I'm building and is full of new interesting technical challenges.
Subscribe to my mailing list to stay up to date on interesting things I overcome and share with everyone.
You can reach me on Twitter if you have any questions or want to connect!