How to Use React's useEffect Hook
Hooks are really, really cool.
They make it easy to do what previously required a decent amount of setup and boilerplate.
I'm going to cover specifically the useEffect
hook, as it's a BIG one and it replaces a lot of boilerplate and memorization.
What is the useEffect hook?
The useEffect
hook is a special hook that lets you perform side effects in your React app.
What this means is you can listen for certain things to happen and perform additional actions on things.
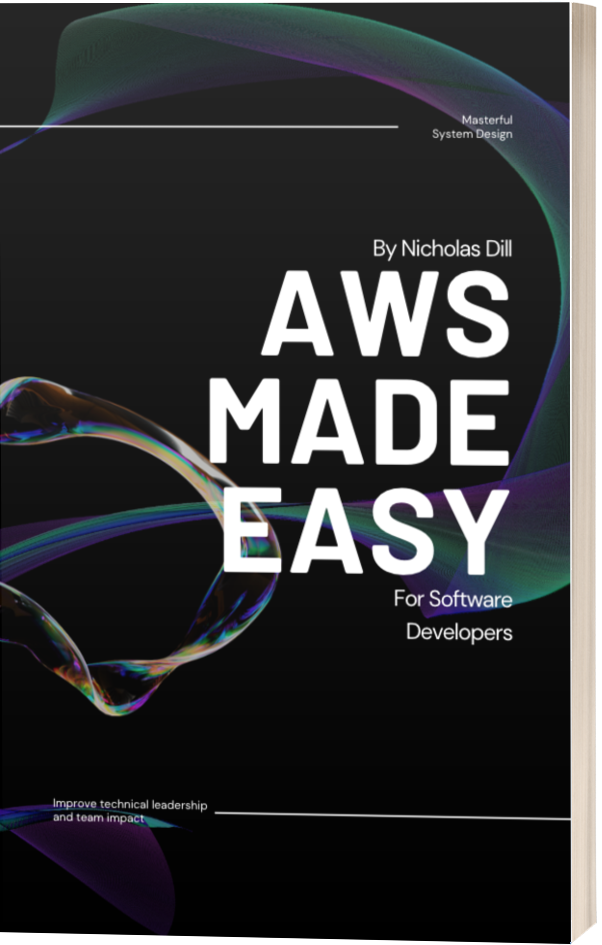
I'll explain with some examples.
How to setup your app with useEffect
First, we need to import this hook to be able to use it. It's pretty straight-forward.
import React, { useEffect } from "react";
Then we call the useEffect function. It will be called on every render of our app.
useEffect
takes 2 arguments:
- a function to perform some logic
- a dependency that tells us if we need to run the function again
Here's an example of this hook in practice. The first argument is a function that prints a string, the second is our dependency.
useEffect(() => {
console.log('called useEffect')
}, [state.count]}
It took me a while to understand how the "dependency" worked but it's easier than it sounds.
Every time we render or re-render, we compare the new value of the dependency to the previous value. If it's different then we call the function we pass as the first argument, and if it's the same value we don't do anything.
In the example above, we pass state.count
as our dependency.
If we update the count then our function will run our console.log('called useEffect')
statement, and if we didn't update the count nothing will happen.
Replacing Lifecycle Methods
Previously, if we wanted certain side effects in our app we would add logic to a number of different lifecycle methods given to us by React.
We used to use the componentWillMount
function which was called whenever our component was going to be mounted in our app.
This can be replaced with the useEffect
hook now.
We can call useEffect
and set our dependency to an empty list.
useEffect(() => {
console.log('called useEffect')
}, []}
Our dependency is just an empty list, which will never change and only run when we first mount the component.
Basically, the first time our component renders run this code but never repeat it when we rerender.
And that's all I got. Hopefully, this helped you understand React hooks a little better.