The Complete Guide to Ruby Comments
Comments are an important part of any programming language. They allow you to communicate with other developers, and explain your code in a way that is easy to understand.
In this guide, we will discuss ruby comments in detail. We will cover how to write comments, how multiline comments work, and how to place comments in ERB files. We will also discuss when to use comments and when not to use them.
Let's get started!
How to Create a Comment in Ruby
At the highest level, comments are lines of code that do not get run with the rest of your program.
You can comment out or insert a comment on any line by beginning the line with a #
.
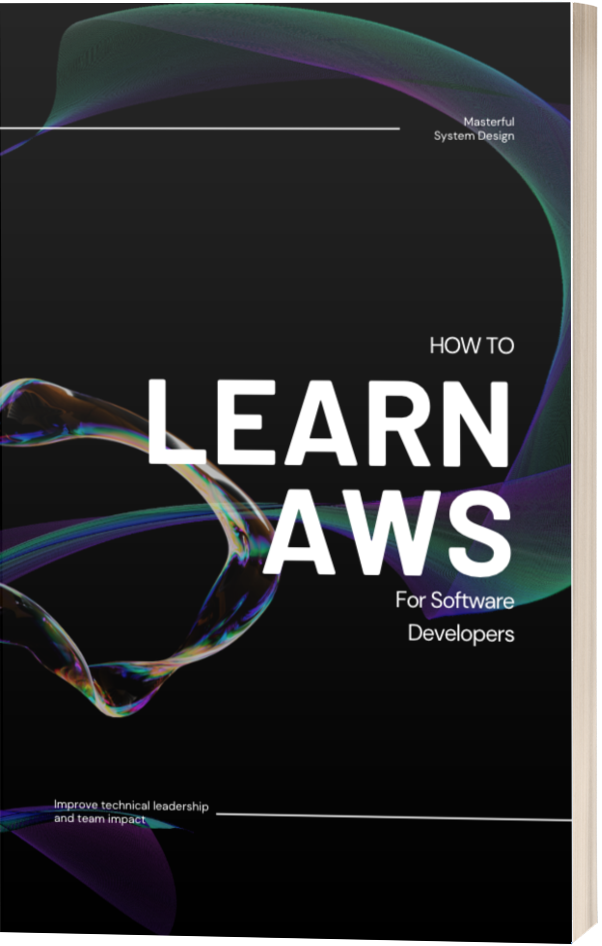
Here is an example of a single line comment:
# This is a single line comment
Traditionally, Ruby developers create multiline comments by stacking single line comments.
# This is a
# multiline comment
# isn't it amazing!
When to Use Comments
Now that we know how to write comments, let's talk about when to use them.
Comments should be used to explain your code. They should not be used to write code that is not self-explanatory. For example, the following code is self-explanatory and does not need a comment:
meaning_of_life = 42 # This is the meaning of life
On the other hand, the following code is not self-explanatory and could use a comment:
x = 42 + (total % 2) # This calculates how many jelly beans we have
In general, you should only write comments when they are truly necessary. Over-commenting your code can make it harder to read and understand.
Comments in ERB View Files
ERB files are used to generate HTML pages from Ruby templates.
They contain both Ruby code and HTML code.
As a result, you have options when it comes to creating comments in these files.
Nothing is stopping you from creating a normal HTML comment, but keep in mind these will be rendered in your site's HTML and savvy visitors will see the comments if they happen to inspect the source code of the page.
<!-- This is an HTML comment. Right-click, select "Inspect element", and you will see the source code includes comments like this one. -->
If you want to hide your comments you should create ERB comments. They look similar but do not make it into the HTML source code for your visitors to see.
<%# This is an ERB comment %>
<%# You just start the line with a # like a normal Ruby comment! %>
Comments in ERB files should be used to explain the purpose of the Ruby code. For example, the following code would be hard to understand without a comment:
<%# This feature is only shown to users with the new feature enabled %>
<% if @user.enabled %>
<p><%= @user.calculate_total %> <%= @user.result %></p>
<% end %>
Takeaways
Comments are an important part of any programming language. They allow you to communicate with other developers, and explain your code in a way that is easy to understand.
In this guide, we have covered Ruby comments in detail. We have discussed how to write comments, how multiline comments work, and how to place comments in ERB files. We have also discussed when to use comments and when not to use them. By following the advice in this guide, you can ensure that your code is well-commented and easy to understand.
# Until next time! Happy coding!