Ruby Templating Engines: ERB vs Haml vs Slim
The Ruby on Rails framework follows the MVC pattern and lets you create views to render the HTML content in your web applications.
These views can be created with plain HTML or with a templating engine.
By default, Rails uses the Embedded Ruby or ERB templating engine. It lets you embed Ruby code directly in your HTML views.
ERB is not the only templating engine though. The other two popular engines are Haml and Slim.
Live dive into what makes them unique and the reasons for picking each one.
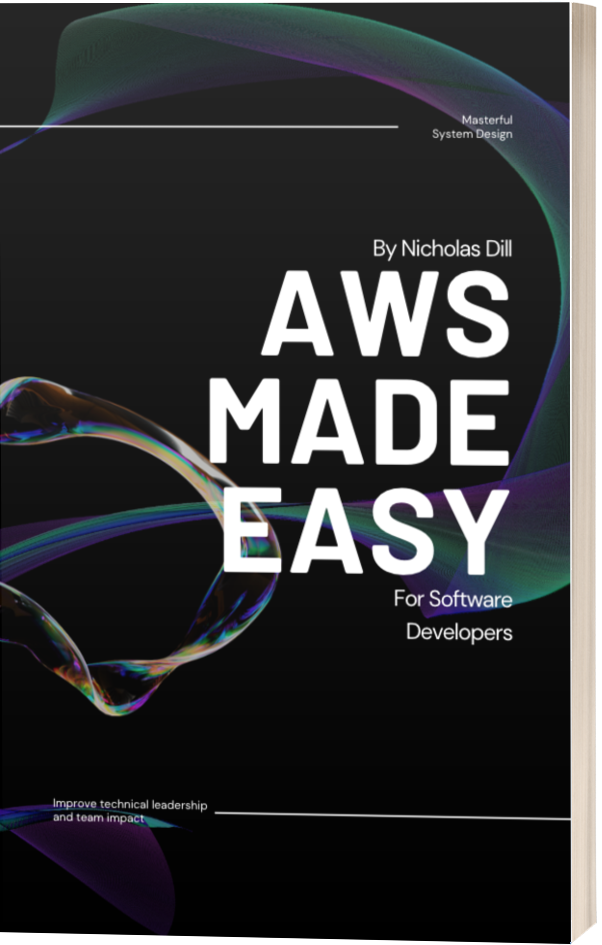
Table of Contents
ERB
I think of ERB as an extension of HTML that gives you access to special <% %>
tags that let you add raw Ruby code to your views.
Your typical index.html
would become index.html.erb
.
This templating engine lets you sprinkle Ruby code into HTML like this:
<div class="title">
<h1><%= @object.name %></h1>
</div>
We use standard HTML syntax, and to insert dynamic Ruby code, you just start a tag with <%
and end it with %>
.
Within these ERB tags we can write normal Ruby code, call variables, functions, classes, and even leave comments that aren't rendered into our HTML document.
Common ERB Tags
Render Output
<%= %>
This will print the output into your HTML. This is why we used the = in the example earlier when we wanted to print the @object.name
inside of our h1.
Again, it could look like this:
<h1><%= @object.name %></h1>
Execute Ruby
<% %>
This will execute the code within the tags. It's great for things like looping over a list of objects and can open and close a block like so:
<% @objects.each do |object| %>
<h1><%= object.name %></h1>
<% end %>
Comments
<%# %>
This tag denotes a comment and will not render anything in your HTML. It matches Ruby's comment syntax by using a #
.
<%# don't forget to use indexes in your database %>
There are a few other tags you can use but this should get you familiar with the basics.
That's really all there is to ERB.
Haml
Haml is named after HTML Abstraction Markup Language.
You would extend the filenames of your views in a way similar to ERB by appending the Haml extension like index.html.haml
.
Otherwise, Haml is quite different from ERB. It really is an abstraction of HTML and unlike ERB, it does not support standard HTML syntax.
If we wanted to create the example above in Haml, it would look like this:
.title
%h1= @object.name
It certainly is less verbose, but clearly looks nothing like HTML.
The HTML output would be unchanged though and would still resemble our original example.
Let's say @object.name
evaluates to Foo
, then the output from this Haml would look like this:
<div class="title">
<h1>Foo</h1>
</div>
An important note about Haml, is that whitespace matters. This templating engine relies on whitespace to determine the structure of your elements. If you mess up the whitespace here, you're gonna have a bad day.
Slim
Slim is the last templating engine to cover. It's similar to Haml in that its syntax does not resemble HTML.
div(class="title")
h1 = @object.name
Slim exists to offer an alternative templating engine that is less verbose than ERB but much faster than Haml.
It also relies on whitespace to evaluate the hierarchy of HTML elements.
The resulting HTML would be the same as the other examples:
<div class="title">
<h1>Foo</h1>
</div>
Performance
ERB is and will always be faster than the alternative templating engines. It wins in every benchmark and does not require any third-party gems.
Every case study will be rendering different code and as a result you will see different results when comparing benchmarks.
Many experiments paint Slim as being about 8x faster than Haml.
But ERB is usually still much faster than Slim.
Pros & Cons
All three of these templating engines are widely used and as a result there is no clear winner. So let's go over the pros and cons for each and talk about the tradeoffs.
ERB
Pros
- fastest parsing performance of all templating engines
- supports plain HTML syntax
- not dependent on whitespace
Cons
- more verbose
- need to be familiar with HTML
Haml
Pros
- DRY and less verbose than ERB
Cons
- slowest performance of all templating engines
- does not support regular HTML syntax
- syntax is whitespace dependent
Slim
Pros
- faster than Haml
- highly configurable
Cons
- slower than ERB
- does not support regular HTML syntax
- syntax is whitespace dependent
So which templating engine is the best?
Ultimately, all three are widely supported and used so it does come down to personal preference and what you value most as a developer and as a team.
When bringing on new developers I would expect more people to be familiar with HTML syntax than Haml or Slim, and so ERB doesn't have as steep of a learning curve.
ERB is also the fastest.
But, Haml and Slim can provide potentially cleaner and safer code. You don't have to dwell on a missing opening or closing bracket. Although whitespace is a whole new problem too.
I think abstracting away HTML with Haml or Slim do provide cleaner-looking concise code, but in the long run the additional learning curve and maintenance make it less appealing as your team grows. So there, I said it. ERB is my favorite.