A Deep Dive into the useMemo Hook in React
I love React hooks and so do you, you just might not know it yet.
What used to require lots of boilerplate and configuring classes can now be accomplished by importing a function called a hook.
Feel free to skip to the useMemo stuff, otherwise...
Quick Overview of Hooks
Hooks let you use new React features like state without having to write classes.
The big idea is that most React functionality can now be imported as functions so there isn't a need to use class-based components.
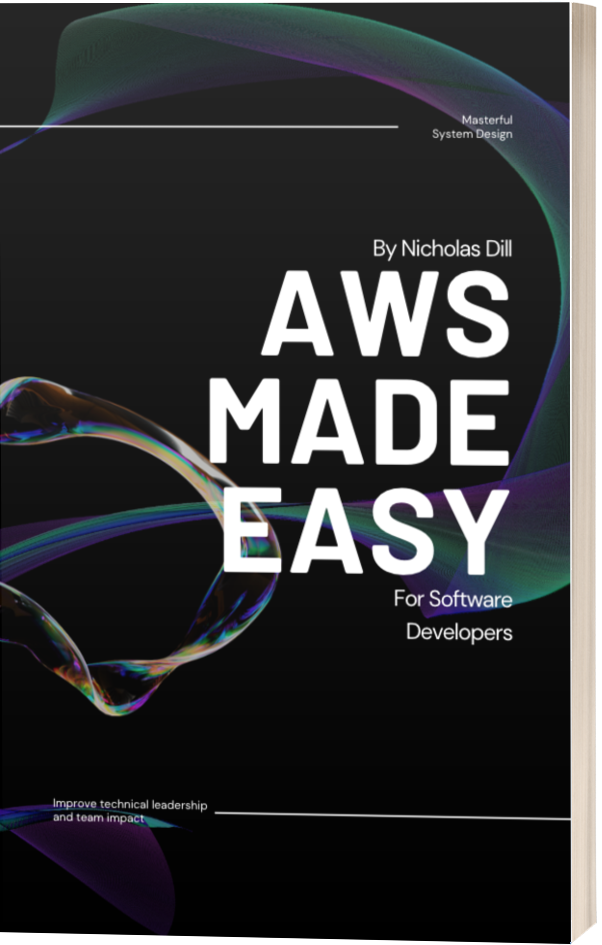
Classes are more complex, confusing, and harder to understand so React is getting away from them and hooks allow you to do that.
Where before with class-based components, you had to define a constructor, initialize the state of the component, and you needed to define a render function just to output the content of the component.
Functional components simplify all of that. Here's an example.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(1);
return (
<div>
<p>The count is {count}</p>
<button onClick={() => setCount(count + 1)}>
Increase
</button>
</div>
);
}
Notice the lack of initializer, thanks to the useState hook. And since this isn't a class we don't have to supply a render method, we just have to return whatever we want this component to render.
Introduction to useMemo
Memoization is a technique used to optimize code by storing the results of function calls and returning this cached result when the function is called with the same inputs again.
The useMemo hook allows you to memoize something in your component such that it does not rerender with the rest of the component unless a specific value changes.
How to Call useMemo
This hook takes 2 arguments.
- The function that we want to cache
- The values that tell us to call the function if they change
It would look like this:
const memoizedResult = useMemo(() => slowFunction(a), [a]);
By default, if your component rendered the result of your slowFunction
above, it would recalculate the value on every single rerender.
That's slow and highly unnecessary.
By wrapping it in the useMemo
hook we tell our React component to save the value between renders and return that saved value unless the value of [a]
changes.